Mybatis generator和tk.Mapper
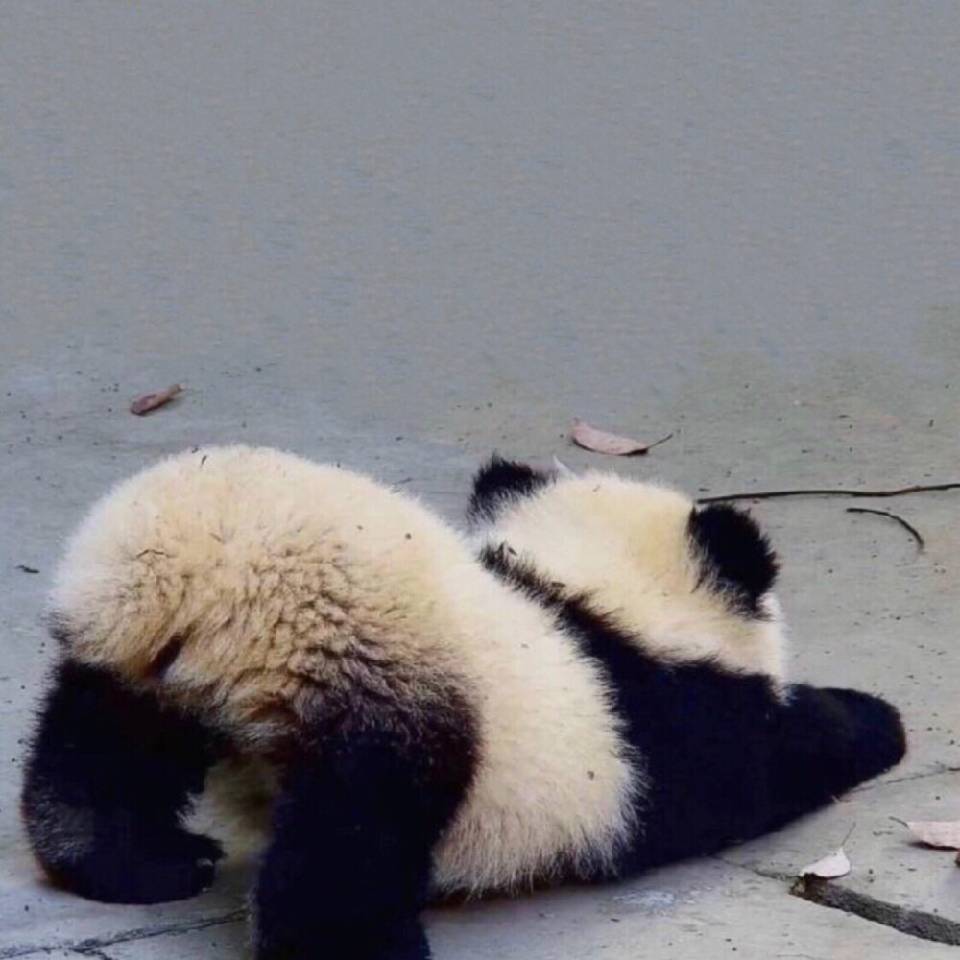
Mybatis generator是什么呢,在使用Mybatis的时候,不仅要自己写entity实体类和dao层,对应的xml文件也要自己去写,不仅工作量很大而且还很枯燥,而Mybatis-generator就是自动生成这些代码的。当然后面发现了更好用的Mapper层接口。
一、Mybatis generator的使用
1.引入 Maven
1 |
|
2.数据库建立
自己随表建一个就行
1 | create table article |
3.generatorConfig.xml文件
这个xml文件的位置对应第一步文件中的位置
1 |
|
需要修改的几个地方
- mysql8版本之后需要加com.mysql.cj.jdbc.Driver。
- entity和mapper的包名改成自己的,路径就是src/main/resources或者src/main/java
- table里面写自己需要生成的表名,后面几个enable都是是否生成对应的example类,column为主键
4.运行
直接点击IDEA右侧的Maven/Plugins/mybatis-generator下面的generator即可,待运行结束,就可以看到自动生成的entity、mapper和xml文件。
二、tk.mybatis.mapper.common.Mapper(通用mapper的使用)
使用
1.引入 Maven
1 | <dependency> |
2.Mapper使用
继承Tk.Mapper,如果不符合业务逻辑的话,可以自己重写
1 | import tk.mybatis.mapper.common.Mapper; |
然后再对应的xml文件里面写增删改查操作
1 |
|
tk.mapper的一些常用方法
本身已经封装了一些基本的单表操作
Base 方法
Select
接口 | 方法 | 说明 |
---|---|---|
SelectMapper |
List |
根据实体中的属性值进行查询,查询条件使用等号 |
SelectByPrimaryKeyMapper |
T selectByPrimaryKey(Object key); | 根据主键字段进行查询,方法参数必须包含完整的主键属性,查询条件使用等号 |
SelectAllMapper |
List |
查询全部结果,select(null)方法能达到同样的效果 |
SelectOneMapper |
T selectOne(T record); | 根据实体中的属性进行查询,只能有一个返回值,有多个结果是抛出异常,查询条件使用等号 |
SelectCountMapper |
int selectCount(T record); | 根据实体中的属性查询总数,查询条件使用等号 |
1 | public Article findArticle(Integer articleId) { |
Insert
接口 | 方法 | 说明 |
---|---|---|
InsertMapper |
int insert(T record); | 保存一个实体,null的属性也会保存,不会使用数据库默认值 |
InsertSelectiveMapper |
int insertSelective(T record); | 保存一个实体,null的属性不会保存,会使用数据库默认值 |
Update
接口 | 方法 | 说明 |
---|---|---|
UpdateByPrimaryKeyMapper |
int updateByPrimaryKey(T record); | 根据主键更新实体全部字段,null值会被更新 |
UpdateByPrimaryKeySelectiveMapper |
int updateByPrimaryKeySelective(T record); | 根据主键更新属性不为null的值 |
Delete
接口 | 方法 | 说明 |
---|---|---|
DeleteMapper |
int delete(T record); | 根据实体属性作为条件进行删除,查询条件使用等号 |
DeleteByPrimaryKeyMapper |
int deleteByPrimaryKey(Object key); | 根据主键字段进行删除,方法参数必须包含完整的主键属性 |
Example 方法
接口 | 方法 | 说明 |
---|---|---|
SelectByExampleMapper |
List |
根据Example条件进行查询,重点:这个查询支持通过Example类指定查询列,通过selectProperties方法指定查询列 |
SelectCountByExampleMapper |
int selectCountByExample(Object example); | 根据Example条件进行查询总数 |
UpdateByExampleMapper |
int updateByExample(@Param(“record”) T record, @Param(“example”) Object example); | 根据Example条件更新实体record包含的全部属性,null值会被更新 |
UpdateByExampleSelectiveMapper |
int updateByExampleSelective(@Param(“record”) T record, @Param(“example”) Object example); | 根据Example条件更新实体record包含的不是null的属性值 |
DeleteByExampleMapper |
int deleteByExample(Object example); | 根据Example条件删除数据 |
1 | public List<Article> findArticleList(Date startCreatTime, Date endCreatTime) { |
RowBounds
默认为内存分页,可以配合PageHelper实现物理分页
接口 | 方法 | 说明 |
---|---|---|
SelectRowBoundsMapper |
List |
根据实体属性和RowBounds进行分页查询 |
SelectByExampleRowBoundsMapper |
List |
根据example条件和RowBounds进行分页查询 |
1 | public List<Article> findArticleList(Integer pageNo, Integer pageSize, String title) { |
- 本文标题:Mybatis generator和tk.Mapper
- 本文作者:Kang
- 创建时间:2022-01-13 16:46:23
- 本文链接:ykhou.github.io2022/01/13/Mybatis-generator的使用/
- 版权声明:本博客所有文章除特别声明外,均采用 BY-NC-SA 许可协议。转载请注明出处!